Microsoft Business Applications Blogposts, YouTube Videos and Podcasts
Helping Businesses with Technology
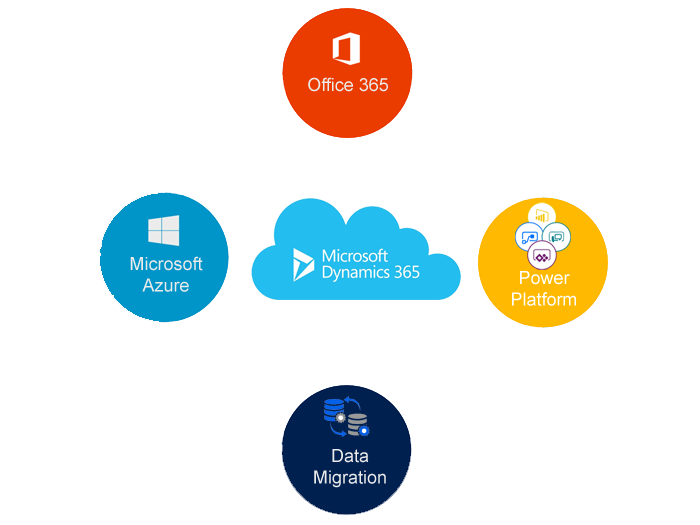
This is a simple windows application to display the methods, properties and constructors using “Reflections” in c#
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Reflection;
namespace ReflectionDemo
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void btnDiscoverTypeInformation_Click_Click(object sender, EventArgs e)
{
string TypeName = txtTypeName.Text;
Type T = Type.GetType(TypeName);
lstMethods.Items.Clear();
lstProperties.Items.Clear();
lstConstructors.Items.Clear();
MethodInfo[] methods = T.GetMethods();
foreach(MethodInfo method in methods)
{
lstMethods.Items.Add(method.ReturnType.Name + ” ” + method.Name);
}
PropertyInfo[] properties = T.GetProperties();
foreach (PropertyInfo property in properties)
{
lstProperties.Items.Add(property.PropertyType.Name + ” ” + property.Name);
}
ConstructorInfo[] constructors = T.GetConstructors();
foreach (ConstructorInfo constructor in constructors)
{
lstConstructors.Items.Add(constructor.ToString());
}
}
}
}